Common Java Coding Mistakes and How to Avoid Them
- Rahul Rana
- Apr 11
- 3 min read
Updated: Apr 12
Java remains one of the most popular programming languages in the world, powering everything from web applications to Android apps and enterprise software. Its versatility and object-oriented nature make it a favorite among developers. However, even experienced Java programmers can fall into common pitfalls that lead to bugs, poor performance, and maintainability issues.
This blog highlights the most frequent Java coding mistakes and provides practical advice on how to avoid them.
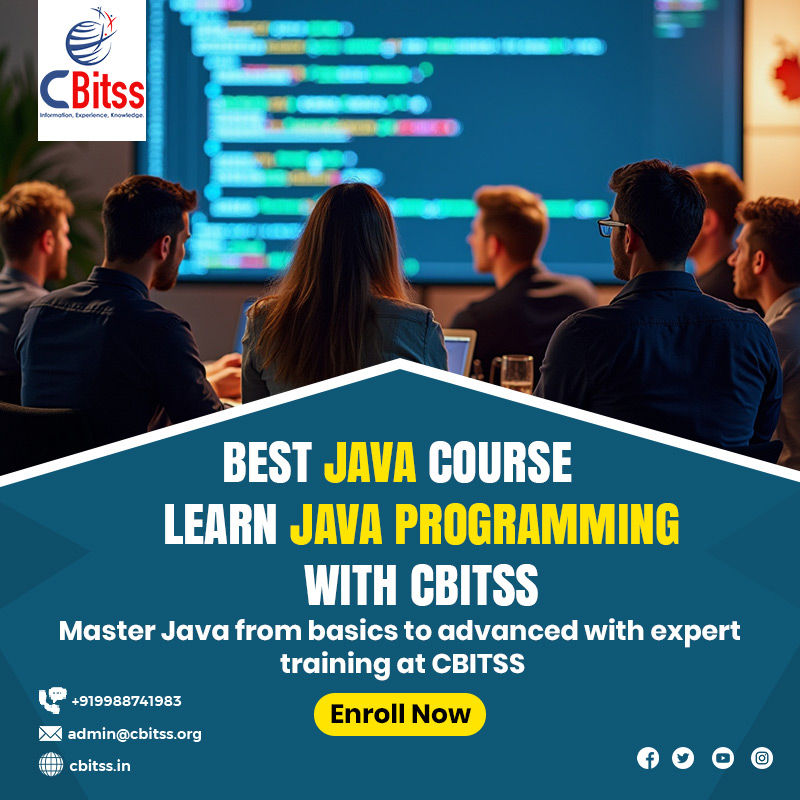
1. Forgetting to Close Resources
Java provides numerous classes for handling external resources like files, database connections, and network sockets. Failing to close these resources can lead to memory leaks and resource exhaustion.
2. Null Pointer Exceptions (NPEs)
Null pointer exceptions are one of the most common runtime errors in Java. They occur when a method tries to use an object reference that hasn’t been initialized.
How to Avoid:
Always perform null checks before accessing object members.
Use Java 8’s Optional class to handle values that might be null.
Avoid returning null from methods where possible.
Optional<String> name = Optional.ofNullable(getUserName());
name.ifPresent(System.out::println);
3. Using == Instead of .equals() for String Comparison
In Java, == checks for reference equality, not content equality. This mistake can lead to unexpected results when comparing strings.
Avoid:
Always use .equals() or .equalsIgnoreCase() for string comparisons.
if (str1.equals(str2)) {
// Correct way to compare strings
}
4. Ignoring Exceptions
Some developers catch exceptions but leave the catch block empty or just print the stack trace without taking action. This approach can hide real problems and make debugging harder.
How to Avoid:
Handle exceptions meaningfully. Log errors, rethrow if necessary, or provide fallback logic.
try {
processFile();
} catch (IOException e) {
logger.error("Failed to process file", e);
}
5. Memory Leaks Due to Unmanaged Collections
Using collections without removing unused objects can cause memory bloat, especially in applications that run continuously.
How to Avoid:
Remove objects from collections when they're no longer needed.
Consider using WeakHashMap for caches to allow garbage collection of unused keys.
6. Overusing Static Variables and Methods
While static methods and variables have their place, overusing them can lead to tightly coupled code and difficulty in testing.
How to Avoid:
Favor instance methods and variables when appropriate. Rely on dependency injection to keep the code flexible and testable.
7. Poor Understanding of Inheritance and Polymorphism
Some developers overuse inheritance or misuse polymorphism, leading to fragile and confusing codebases.
How to Avoid:
Prefer composition over inheritance.
Use interfaces to define behavior and keep your classes loosely coupled.
8. Improper Use of Collections
Choosing the wrong collection type or modifying collections while iterating can lead to runtime exceptions like ConcurrentModificationException.
How to Avoid:
Understand the differences between ArrayList, LinkedList, HashMap, HashSet, etc.
Use Iterator’s remove() method if you need to modify collections during iteration.
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
if (someCondition) {
iterator.remove(); // Safe removal
}
}
9. Neglecting Synchronization in Multithreaded Code
Java makes it easy to write multithreaded applications, but failing to synchronize shared resources can lead to data inconsistency.
How to Avoid:
Use synchronization blocks or ReentrantLock where appropriate.
For collections, use concurrent data structures like ConcurrentHashMap.
synchronized (this) {
// thread-safe block
}
10. Not Following Java Naming Conventions
Naming conventions improve code readability and maintainability. Ignoring them may not break the code, but it does affect collaboration and professional standards.
How to Avoid:
Class names should be in PascalCase (e.g., CustomerManager)
Method and variable names should be in camelCase (e.g., calculateTotalPrice)
Avoiding common Java mistakes starts with awareness and continuous learning. Whether you're a beginner or a seasoned developer, writing clean, efficient, and bug-free Java code is a discipline that improves with practice. By understanding these pitfalls and applying the best practices discussed above, you can boost the quality of your code and become a more effective Java developer
Key Takeaways:
Use try-with-resources to manage external resources
Avoid NullPointerException with null checks or Optional
Always compare strings with .equals()
Handle exceptions responsibly
Use the right data structures and synchronization techniques
Follow Java conventions to write professional code
Commenti